编程跳一跳小游戏
- 游戏相关
- 2024-09-20 06:26:01
跳一跳小游戏编程
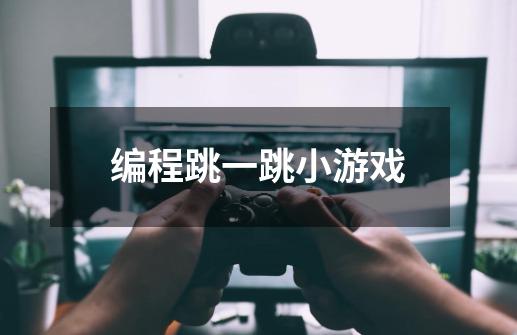
简介
跳一跳是一款风靡全球的休闲小游戏,其玩法简单,却极具挑战性。玩家需要控制一个方块在不断移动的平台上跳跃,目标是尽可能跳得更远。本文将介绍如何使用 HTML 和 JavaScript 编程跳一跳小游戏。游戏环境
创建游戏环境需要一个 HTML 页面和一个 JavaScript 文件。HTML 页面定义游戏画布,而 JavaScript 文件包含游戏逻辑。 ```html ```画布设置
画布是游戏的视觉显示区域。使用 JavaScript 设置画布大小和背景色。 ```javascript // 获取画布 const canvas = document.getElementById('canvas'); // 获取画布上下文 const ctx = canvas.getContext('2d'); // 设置画布大小 canvas.width = 500; canvas.height = 300; // 设置背景色 ctx.fillStyle = 'white'; ctx.fillRect(0, 0, canvas.width, canvas.height); ```方块对象
方块是玩家控制的角色。创建一个方块对象,并定义其属性和方法。 ```javascript class Block { constructor(x, y, width, height) { this.x = x; this.y = y; this.width = width; this.height = height; this.speedY = 0; this.isJumping = false; } render() { ctx.fillStyle = 'black'; ctx.fillRect(this.x, this.y, this.width, this.height); } update() { if (this.isJumping) { this.speedY -= 0.1; } this.y -= this.speedY; // 落地检测 if (this.y + this.height >= canvas.height) { this.speedY = 0; this.isJumping = false; this.y = canvas.height - this.height; } } } ```平台对象
平台是方块跳跃的目标。创建一个平台对象,并定义其属性和方法。 ```javascript class Platform { constructor(x, y, width, height) { this.x = x; this.y = y; this.width = width; this.height = height; } render() { ctx.fillStyle = 'green'; ctx.fillRect(this.x, this.y, this.width, this.height); } } ```游戏逻辑
游戏逻辑控制游戏的进行,包括方块的跳跃、平台的移动等。 ```javascript // 方块对象 let block = new Block(100, canvas.height - 100, 20, 20); // 平台对象 let platforms = []; for (let i = 0; i < 10; i++) { platforms.push(new Platform(i * 50, 100 + i * 50, 20, 10)); } // 游戏主循环 function gameLoop() { // 清除画布 ctx.clearRect(0, 0, canvas.width, canvas.height); // 更新方块 block.update(); // 更新平台 for (let platform of platforms) { platform.render(); } // 渲染方块 block.render(); // 检测方块与平台的碰撞 for (let platform of platforms) { if (block.x + block.width >= platform.x && block.x <= platform.x + platform.width && block.y + block.height >= platform.y && block.y <= platform.y + platform.height) { block.isJumping = false; block.speedY = 0; block.y = platform.y - block.height; } } // 请求下一次动画帧 requestAnimationFrame(gameLoop); } // 启动游戏主循环 gameLoop(); ```按键响应
添加按键响应,使玩家可以通过键盘控制方块的跳跃。 ```javascript addEventListener('keydown', e => { if (e.key === 'Space') { block.isJumping = true; block.speedY = 5; } }); ```总结
通过以上步骤,我们就可以使用 HTML 和 JavaScript 编程出跳一跳小游戏。该游戏包含了方块的移动、平台的刷新、碰撞检测等基本游戏元素。通过扩展游戏逻辑,还可以添加更多功能,如积分计算、排行榜等,打造更丰富的游戏体验。上一篇
维森游戏植物大战僵尸
下一篇
网游2024排行榜前十名